In the realm of machine learning and image processing, latent space representations play a crucial role in understanding and manipulating complex data. Latent space, in this context, refers to a compressed and often lower-dimensional representation of data, typically images, that captures the most salient features. Converting images from latent space back into pixel space is a critical step in various applications, including image generation, data compression, and image synthesis. This article delves into the process of this conversion, its significance, and the detailed mechanics behind it.
Understanding Latent Space
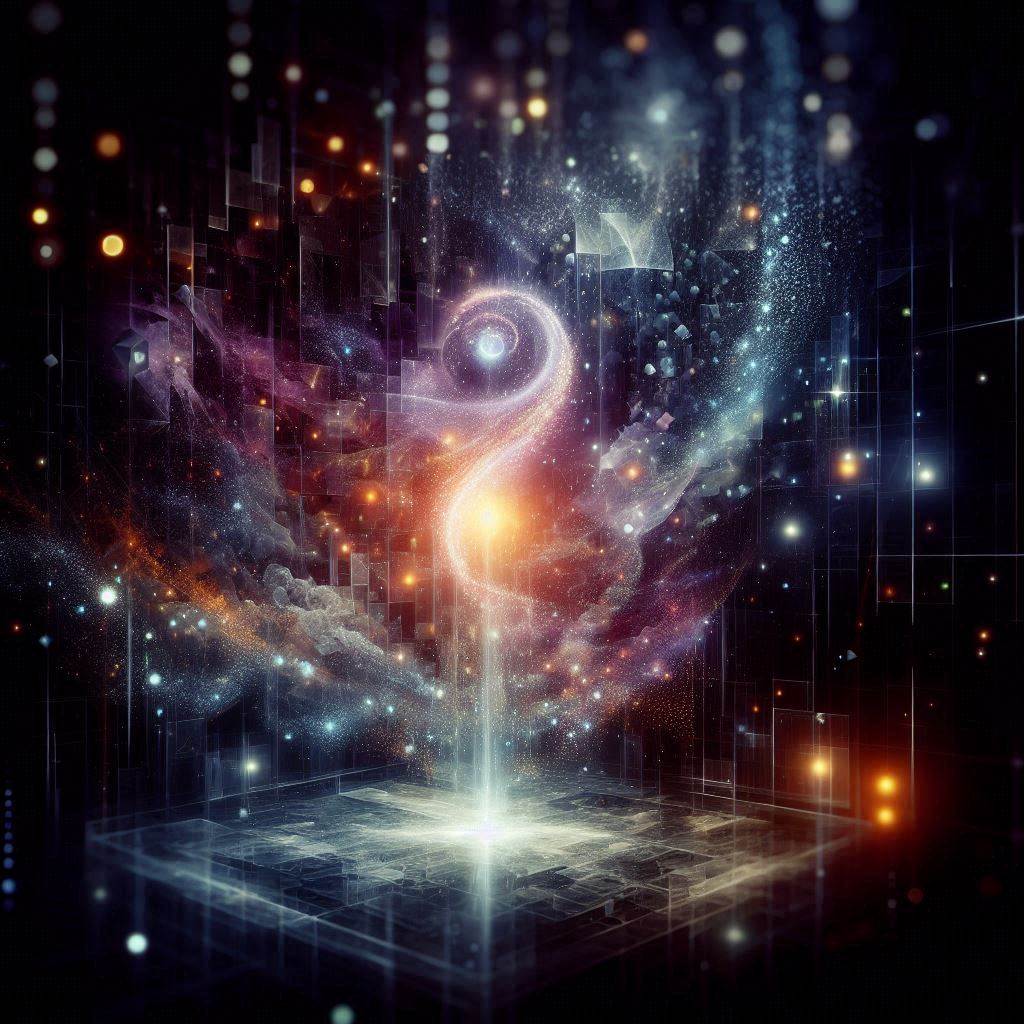
Latent space is a concept used in many machine learning models, particularly in autoencoders and generative adversarial networks (GANs). When an image is encoded into latent space, it is transformed into a lower-dimensional vector that encodes the essential features of the image while discarding redundant information. This transformation is achieved using an encoder network in autoencoders or the generator network in GANs.
Why Convert Back to Pixel Space?
Converting latent space representations back into pixel space is necessary for several reasons:
- Image Generation: In generative models like GANs, the latent space is sampled to create new images. These images are in latent space and need to be converted back to pixel space to be visually interpreted.
- Data Compression: Autoencoders compress data into latent space for efficient storage or transmission. To reconstruct the original data, the latent representation must be decoded back to pixel space.
- Image Manipulation: Latent space representations allow for manipulation of images at a feature level, such as altering facial expressions or styles. These manipulations are meaningful only when converted back to pixel space.
- Anomaly Detection: In tasks like anomaly detection, latent space representations of normal images are compared to those of new images. Reconstruction in pixel space is essential to identify and visualize anomalies.
The Process of Conversion
The process of converting a latent space image back into pixel space typically involves a decoder network, which is designed to perform the inverse operation of the encoder. Here’s a detailed step-by-step explanation of this process:
- Encoding Phase (Latent Space Representation):
- Input Image: The original image in pixel space is fed into the encoder network.
- Feature Extraction: The encoder compresses the image into a set of feature maps through a series of convolutional layers, pooling, and non-linear activations.
- Latent Vector: The final output of the encoder is a latent vector, a compact representation of the input image.
- Decoding Phase (Reconstruction in Pixel Space):
- Latent Vector Input: The latent vector is fed into the decoder network.
- Upsampling and Deconvolution: The decoder performs upsampling and deconvolution operations to expand the latent vector back to the original image dimensions. This involves transposed convolutions or other upsampling techniques that gradually reconstruct the spatial dimensions.
- Feature Reconstruction: Through a series of layers, the decoder reconstructs the high-dimensional pixel information from the low-dimensional latent representation. This process often mirrors the operations performed by the encoder but in reverse.
- Output Image: The final output of the decoder is an image in pixel space, which ideally resembles the original input image.
Practical Implementation
Let’s consider an example using a convolutional autoencoder. Here is a simplified Python implementation using TensorFlow and Keras:
import tensorflow as tf
from tensorflow.keras.layers import Input, Conv2D, MaxPooling2D, UpSampling2D
from tensorflow.keras.models import Model
# Encoder
input_img = Input(shape=(28, 28, 1)) # Example for MNIST dataset
x = Conv2D(32, (3, 3), activation='relu', padding='same')(input_img)
x = MaxPooling2D((2, 2), padding='same')(x)
x = Conv2D(64, (3, 3), activation='relu', padding='same')(x)
latent = MaxPooling2D((2, 2), padding='same')(x)
# Decoder
x = Conv2D(64, (3, 3), activation='relu', padding='same')(latent)
x = UpSampling2D((2, 2))(x)
x = Conv2D(32, (3, 3), activation='relu', padding='same')(x)
x = UpSampling2D((2, 2))(x)
decoded_img = Conv2D(1, (3, 3), activation='sigmoid', padding='same')(x)
# Autoencoder Model
autoencoder = Model(input_img, decoded_img)
autoencoder.compile(optimizer='adam', loss='binary_crossentropy')
# Summary of the model
autoencoder.summary()
In this example, the encoder compresses the input image to a latent vector, and the decoder reconstructs the image from this latent vector. The
UpSampling2D
and
Conv2D
layers in the decoder perform the conversion from latent space back to pixel space.
Challenges and Considerations
- Loss of Information: Perfect reconstruction is often challenging due to information loss during encoding. The design of the network and loss function is crucial to minimize this loss.
- Dimensionality: The choice of latent space dimensionality affects the quality of reconstruction. Too small a latent space might miss essential features, while too large might not offer significant compression benefits.
- Training Stability: Training autoencoders or GANs can be unstable. Techniques like batch normalization, dropout, and careful design of the architecture help in achieving stable training and better reconstructions.